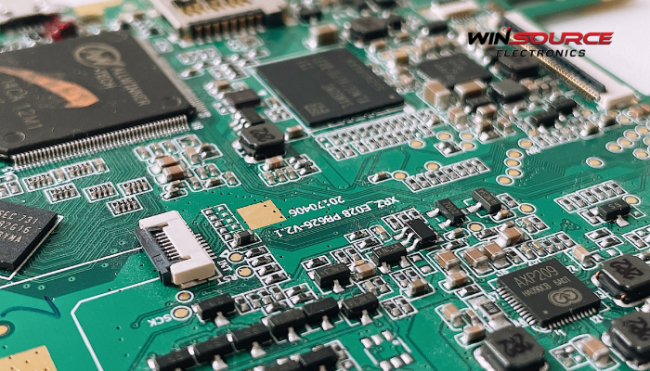
* Question
Which registers are controlled by each pin that is a GPIo function?
* Answer
When a pin on a microcontroller or other programmable device is configured as a GPIO (General Purpose Input/Output), the specific registers controlling its behavior typically include:
1. Direction Register (DIR or similar)
– Determines whether the pin is configured as an input or an output.
– Example: `GPIOx_DIR` (1 for output, 0 for input).
2. Output Register (OUT or similar)
– Used to write a high or low signal to the pin when configured as an output.
– Example: `GPIOx_OUT`.
3. Input Register (IN or similar)
– Used to read the state of the pin when configured as an input.
– Example: `GPIOx_IN`.
4. Pull-up/Pull-down Control Register (PUPD or similar)
– Configures the internal pull-up or pull-down resistors for the pin when it is set as an input.
– Example: `GPIOx_PUPDR`.
5. Alternate Function Select Register (AFSEL or similar)
– Determines whether the pin operates as a GPIO or an alternate peripheral function.
– Example: `GPIOx_AFSEL`.
6. Drive Strength Control Register (DRIVE or similar)
– Configures the drive strength (current capacity) of the pin when driving an output.
– Example: `GPIOx_DRIVE`.
7. Interrupt Configuration Register
– Controls interrupt behavior, such as edge triggering (rising/falling edge or level).
– Example: `GPIOx_IM` (Interrupt Mask), `GPIOx_IEV` (Interrupt Event), `GPIOx_ICR` (Interrupt Clear).
8. Mode Register (MODER or similar)
– Configures the mode of the pin (e.g., input, output, alternate function, analog).
– Example: `GPIOx_MODER`.
9. Lock Register (LOCK or similar)
– Locks the configuration of the GPIO pin to prevent accidental changes.
– Example: `GPIOx_LOCK`.
10. Analog/Digital Configuration Register
– Configures whether the pin operates in analog or digital mode.
– Example: `GPIOx_AMSEL`.
11. Debounce Control Register
– Configures debounce settings for the pin (if supported).
– Example: `GPIOx_DEBOUNCE`.
12. Slew Rate Control Register
– Configures the slew rate (rate of change of the signal) for the pin.
– Example: `GPIOx_SR`.
The exact naming and availability of these registers depend on the microcontroller or device family (e.g., STM32, Arduino/AVR, PIC, ESP32). You should consult the datasheet or reference manual for your specific microcontroller to determine the exact registers for controlling GPIO pins.
COMMENTS