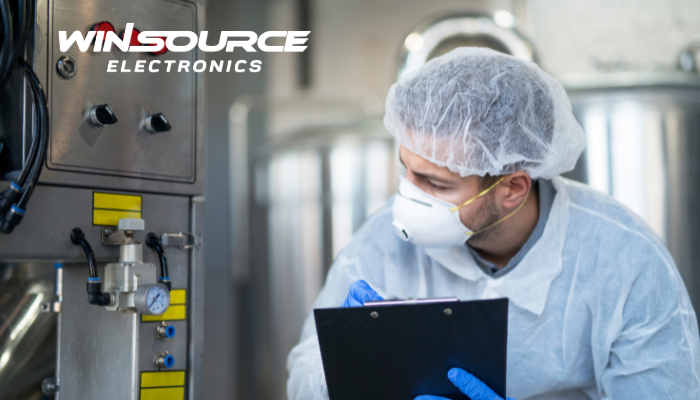
* Question
How to generate temperature error?
* Answer
Generating temperature error for testing or calibration purposes involves creating a controlled deviation from the actual temperature. Here are some methods to generate and manage temperature error in various contexts:
1. Simulating Temperature Error in Software
– Offset Addition: Introduce a fixed offset to the actual temperature readings. For example, if the actual temperature is 25°C, adding a 2°C offset would simulate a reading of 27°C.
“`python
actual_temperature = 25.0 # Actual temperature in degrees Celsius
error_offset = 2.0 # Error offset in degrees Celsius
simulated_temperature = actual_temperature + error_offset
print(simulated_temperature) # Output will be 27.0
“`
– Random Noise: Add random noise to the temperature readings to simulate measurement errors.
“`python
import random
actual_temperature = 25.0 # Actual temperature in degrees Celsius
error_range = (-0.5, 0.5) # Range of random error in degrees Celsius
simulated_temperature = actual_temperature + random.uniform(*error_range)
print(simulated_temperature) # Output will vary within the range
“`
2. Using Hardware for Temperature Error Generation
– Temperature Calibration Devices: Use devices specifically designed for calibrating temperature sensors. These devices can generate controlled temperature errors.
– Thermal Chambers: Place the temperature sensor in a thermal chamber and manipulate the chamber settings to create a known temperature discrepancy.
– Resistor Substitution: In temperature measurement circuits, use resistors with known inaccuracies to simulate sensor errors. For example, if an RTD (Resistance Temperature Detector) is used, replace it with a resistor of slightly different resistance to simulate a temperature error.
3. Manual Methods
– Insulation Variation: Modify the insulation around the temperature sensor to create a known error due to thermal lag or thermal gradients.
– Physical Displacement: Place the sensor in a location where the temperature is known to be different from the desired measurement point, thereby creating a controlled discrepancy.
4. Environmental Simulation
– Controlled Environment Changes: Create a controlled environment where the actual temperature is known, and then use a faulty or miscalibrated sensor to generate errors. This can be done in an environmental testing chamber where temperature is precisely controlled and monitored.
Example Scenario: Testing a Temperature Monitoring System
Assume you need to test a temperature monitoring system that should trigger an alert if the temperature deviates from a set point (e.g., 25°C) by more than 2°C.
1. Software Simulation:
“`python
import random
actual_temperature = 25.0 # Actual temperature in degrees Celsius
error_offset = 3.0 # Error to trigger the alert
simulated_temperature = actual_temperature + error_offset
if abs(simulated_temperature – actual_temperature) > 2.0:
print(“Alert: Temperature deviation detected!”) # This should trigger the alert
else:
print(“Temperature is within acceptable range.”)
“`
2. Using a Thermal Chamber:
– Set the chamber to 25°C.
– Introduce a known heat source or cooling element to create a 3°C deviation.
– Observe if the monitoring system triggers the alert as expected.
Generating temperature errors can be achieved through software simulations, using hardware devices, or creating controlled environmental conditions. Each method depends on the context of the test and the precision required. These techniques help ensure that temperature monitoring and control systems are robust and accurate under various conditions.
COMMENTS