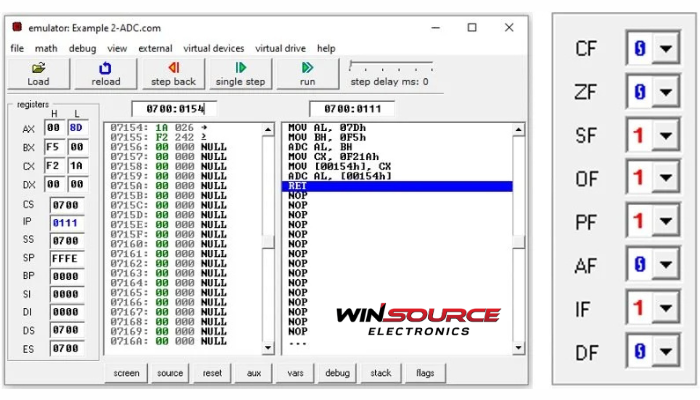
* Question
What are the arithmetic operation instructions?
* Answer
Arithmetic operation instructions are a subset of instructions in a processor’s instruction set that perform basic mathematical operations on data. These instructions are essential for manipulating numerical values and are found in virtually all computer architectures. The types and availability of these instructions may vary depending on the specific processor or instruction set architecture (ISA).
Here are the common types of arithmetic operation instructions:
1. Addition Instructions
– Purpose: Add two operands.
– Examples:
– `ADD`: Adds two numbers and stores the result.
– `ADDI`: Adds an immediate value to a register (common in RISC architectures).
– `ADC` (Add with Carry): Adds two numbers and includes the carry bit from a previous operation.
2. Subtraction Instructions
– Purpose: Subtract one operand from another.
– Examples:
– `SUB`: Subtracts one number from another.
– `SUBI`: Subtracts an immediate value from a register.
– `SBC` (Subtract with Carry): Subtracts two numbers and includes the carry/borrow bit.
3. Multiplication Instructions
– Purpose: Multiply two operands.
– Examples:
– `MUL`: Performs unsigned multiplication.
– `IMUL`: Performs signed multiplication.
– `MULU`: Multiplication for unsigned numbers (in some architectures).
4. Division Instructions
– Purpose: Divide one operand by another.
– Examples:
– `DIV`: Performs unsigned division.
– `IDIV`: Performs signed division.
– Additional Features:
– Quotient and remainder are often stored in specific registers.
5. Increment and Decrement Instructions
– Purpose: Increase or decrease a value by 1.
– Examples:
– `INC`: Increments the value of a register or memory location by 1.
– `DEC`: Decrements the value of a register or memory location by 1.
6. Negation Instructions
– Purpose: Compute the two’s complement (negate) of an operand.
– Examples:
– `NEG`: Negates a value (e.g., `NEG R1` sets `R1 = -R1`).
7. Absolute Value Instructions
– Purpose: Compute the absolute value of a signed operand.
– Examples:
– `ABS`: Returns the absolute value of an operand (in some ISAs).
8. Comparison Instructions
– Purpose: Subtract two operands without storing the result, used to set condition flags for decision-making.
– Examples:
– `CMP`: Compares two values by subtracting one from the other.
– `CMPI`: Compares a register with an immediate value.
– `TEST`: Performs a bitwise AND to set condition flags (used in logical comparisons).
9. Bit Field Manipulation (Arithmetic Context)
– Purpose: Manipulate specific bit fields relevant to arithmetic operations.
– Examples:
– `BSET`, `BCLR`: Set or clear specific bits in registers.
– `SHL`, `SHR` (Shift left/right): Can be considered arithmetic when used for multiplication/division by powers of 2.
10. Extended Arithmetic Instructions
– Purpose: Perform more advanced operations.
– Examples:
– `DIVIDE/MOD`: Separate instructions for division and modulus operations.
– `SQRT`: Compute the square root (common in DSP and floating-point units).
– `CARRY`, `OVERFLOW` Instructions: Handle special arithmetic conditions.
11. Floating-Point Arithmetic Instructions
– Purpose: Perform arithmetic on floating-point numbers (requires a floating-point unit, FPU).
– Examples:
– `FADD`: Floating-point addition.
– `FSUB`: Floating-point subtraction.
– `FMUL`: Floating-point multiplication.
– `FDIV`: Floating-point division.
Common Use Cases:
– Basic Math: General-purpose addition, subtraction, multiplication, and division.
– Signal Processing: Multiply-accumulate operations in DSPs.
– Conditional Logic: Setting flags for branching and decision-making.
– Scientific Computation: Floating-point arithmetic for precision.
By leveraging these instructions, processors can perform both simple and complex mathematical operations efficiently.
COMMENTS