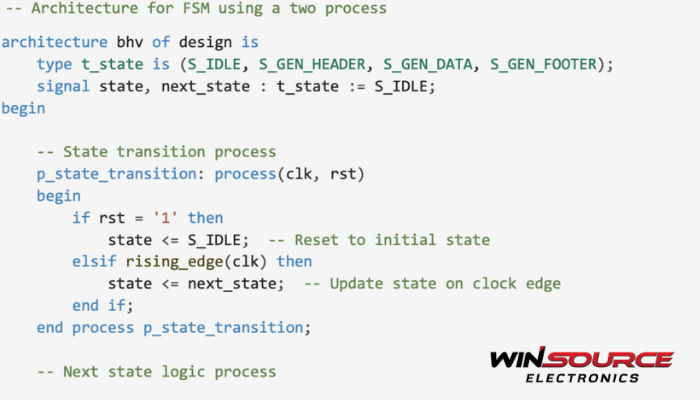
* Question
What are the specific steps for applying a VHDL design state machine?
* Answer
When applying a state machine in VHDL (VHSIC Hardware Description Language), the goal is to
create a digital system that transitions between different states based on inputs and time. A
typical implementation involves several key steps:
Step 1: Define the Problem and Identify States
– Understand the Requirements: Start by clearly defining the problem or the behavior you want
to model. Identify all the possible states the system can be in and determine the conditions or
inputs that will trigger transitions between these states.
– State Diagram: Draw a state diagram that visually represents all states, transitions, inputs, and
outputs. This helps in organizing the logic before coding.
Step 2: Declare State Types and Signals in VHDL
– Define State Enumeration: In VHDL, states are typically defined using an enumerated type.
This allows the states to be represented symbolically (e.g., `IDLE`, `RUN`, `STOP`) rather than
using numeric values.
“`vhdl
type state_type is (IDLE, RUN, STOP);
signal current_state, next_state : state_type;
“`
– Declare Necessary Signals: Besides the state signals, declare other input, output, and internal
signals that will be used in the state machine.
Step 3: Create the State Machine Process
The state machine logic is usually created inside one or more `process` blocks in VHDL. A typical
finite state machine (FSM) implementation includes three parts:
– State Register: To update the current state based on the next state.
– Next State Logic: To define the transitions between states based on inputs.
– Output Logic: To determine the outputs depending on the current state.
The process can be organized as follows:
1. State Register: Create a synchronous process that updates the `current_state` on each clock
edge.
“`vhdl
process (clk, reset)
begin
if reset = ‘1’ then
current_state <= IDLE;
elsif rising_edge(clk) then
current_state <= next_state;
end if;
end process;
“`
2. Next State Logic: Determine the transitions between states based on the current state and
input signals.
“`vhdl
process (current_state, input_signal)
begin
case current_state is
when IDLE =>
if start = ‘1’ then
next_state <= RUN;
else
next_state <= IDLE;
end if;
when RUN =>
if stop = ‘1’ then
next_state <= STOP;
else
next_state <= RUN;
end if;
when STOP =>
if reset = ‘1’ then
next_state <= IDLE;
else
next_state <= STOP;
end if;
when others =>
next_state <= IDLE;
end case;
end process;
“`
3. Output Logic: Define outputs based on the current state and inputs. This can be included in the
same process or in a separate combinational process.
“`vhdl
process (current_state)
begin
case current_state is
when IDLE =>
output_signal <= ‘0’;
when RUN =>
output_signal <= ‘1’;
when STOP =>
output_signal <= ‘0’;
when others =>
output_signal <= ‘0’;
end case;
end process;
“`
Step 4: Simulation and Testing
– Testbench Creation: Write a testbench in VHDL to simulate your state machine design. The
testbench should apply different input sequences and observe the state transitions and outputs.
– Simulation: Run the simulation using a VHDL simulator (such as ModelSim, Vivado, or Quartus)
to verify that your design works as intended. Check the waveform and debug any issues that
arise.
Step 5: Synthesis and Implementation
– Synthesize the Design: Use a synthesis tool to generate a gate-level netlist for your state
machine. Verify that the synthesized design matches the intended state machine behavior.
– Place and Route: If your design is to be implemented on FPGA, use place-and-route tools to
generate the bitstream for programming the FPGA.
Step 6: Hardware Testing
– Program the FPGA: Load the bitstream onto the FPGA and test the design in real hardware, if
applicable. Validate the functionality and performance of your state machine in the target
environment.
Summary
1. Define the problem and identify states.
2. Declare state types and signals in VHDL.
3. Create the state machine process:
– State register (sequential process).
– Next state logic (combinational process).
– Output logic (combinational process).
4. Simulate and test with a testbench.
5. Synthesize and implement the design.
6. Program and test on hardware (if applicable).
These steps provide a structured approach to implementing a state machine in VHDL, ensuring a
clear understanding of the design, development, and testing processes.
COMMENTS