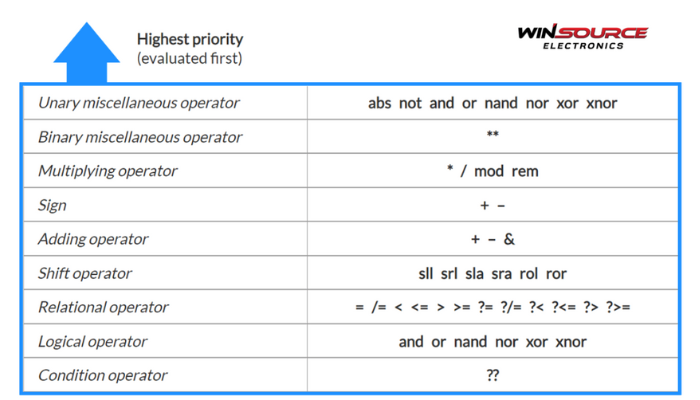
* Question
What are the logical operators in VHDL?
* Answer
In VHDL (VHSIC Hardware Description Language), logical operators are used to perform Boolean operations on signals or variables. These operators are commonly applied in digital design to manipulate `std_logic`, `bit`, and `boolean` data types. Here are the primary logical operators in VHDL:
1. AND
– Performs a logical AND operation between two operands. The result is `TRUE` only if both operands are `TRUE` (or ‘1’ in `std_logic`).
Example:
“`vhdl
signal a, b, result: std_logic;
result <= a AND b; — result is ‘1’ if both a and b are ‘1’
“`
2. OR
– Performs a logical OR operation between two operands. The result is `TRUE` if at least one operand is `TRUE`.
Example:
“`vhdl
signal a, b, result: std_logic;
result <= a OR b; — result is ‘1’ if either a or b is ‘1’
“`
3. NAND
– Performs a NOT AND operation. It is the negation of the `AND` operator. The result is `TRUE` unless both operands are `TRUE`.
Example:
“`vhdl
signal a, b, result: std_logic;
result <= a NAND b; — result is ‘1’ unless both a and b are ‘1’
“`
4. NOR
– Performs a NOT OR operation. It is the negation of the `OR` operator. The result is `TRUE` only if both operands are `FALSE`.
Example:
“`vhdl
signal a, b, result: std_logic;
result <= a NOR b; — result is ‘1’ only if both a and b are ‘0’
“`
5. XOR
– Performs an exclusive OR operation. The result is `TRUE` if one operand is `TRUE` and the other is `FALSE`.
Example:
“`vhdl
signal a, b, result: std_logic;
result <= a XOR b; — result is ‘1’ if one of a or b is ‘1’, but not both
“`
6. XNOR
– Performs an exclusive NOR operation, which is the inverse of `XOR`. The result is `TRUE` if both operands are either `TRUE` or `FALSE`.
Example:
“`vhdl
signal a, b, result: std_logic;
result <= a XNOR b; — result is ‘1’ if both a and b are the same (both ‘1’ or both ‘0’)
“`
7. NOT
– Performs a logical NOT operation, which inverts the value of the operand. If the operand is `TRUE`, the result will be `FALSE`, and vice versa.
Example:
“`vhdl
signal a, result: std_logic;
result <= NOT a; — result is ‘1’ if a is ‘0’, and vice versa
“`
Application:
These operators are widely used in VHDL to define the behavior of combinational logic circuits, such as gates, multiplexers, and arithmetic units, as well as in the conditional control of sequential logic.
Logical operators in VHDL can be applied to various data types like `boolean`, `bit`, `std_logic`, and `std_logic_vector`, making them essential in hardware description and design.
COMMENTS